Unidirectional Data Flow as a Functional declaration
Representing UI state production pipelines as a function of a stream of inputs yielding a stream of output states
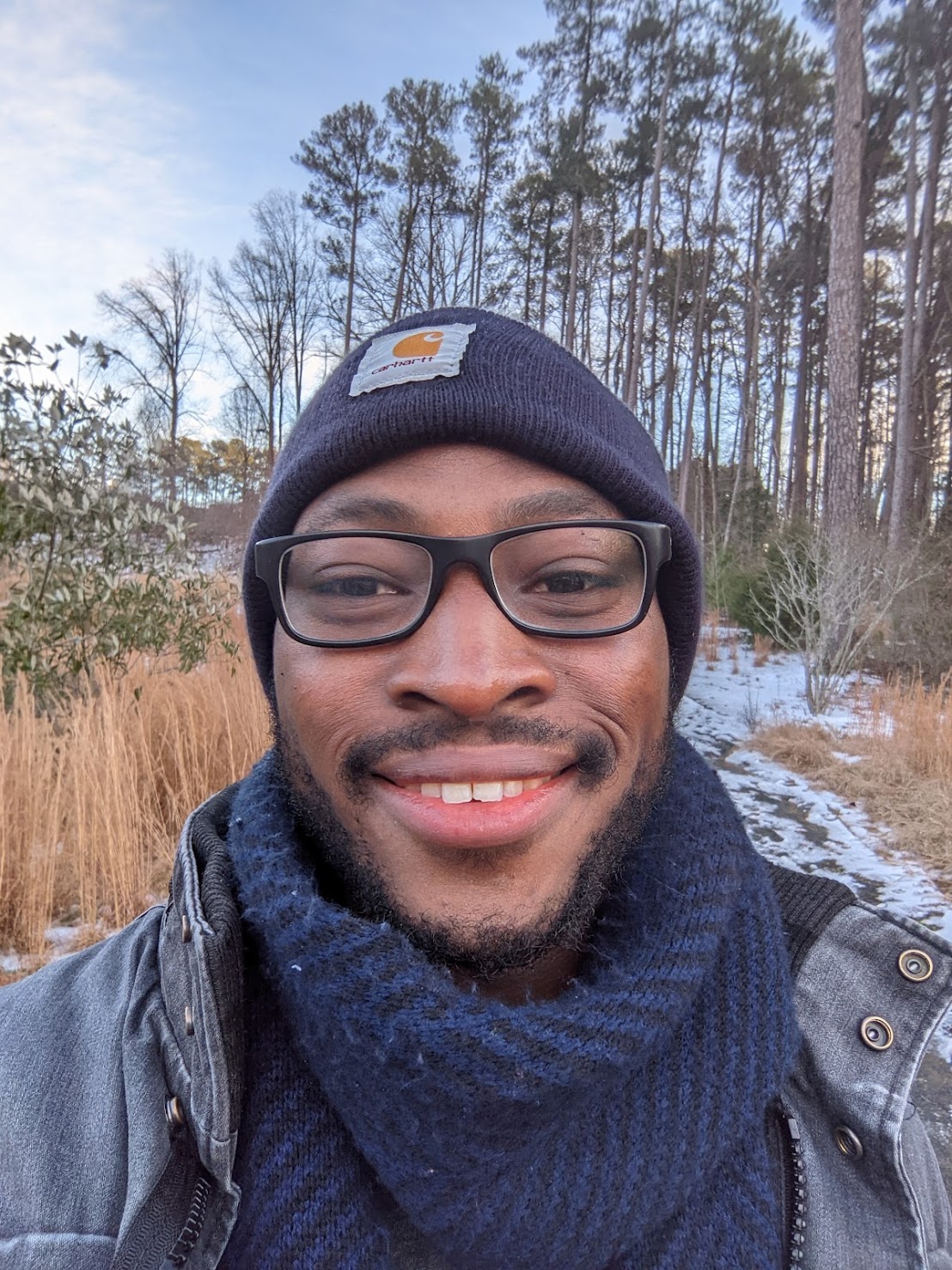
TJ Dahunsi
Mar 15 2022 · 12 mins
There are a lot of interesting experiments and learnings from the android community about the production of UI state in the context of a Unidirectional Data Flow (UDF) app. Like with most things, while the “what”, in this case UDF, may be well defined, “how” is quite the moving target.
This article will briefly cover some approaches on the topic, and then introduce my personal take on it. Extra emphasis on personal, my work isn't peer reviewed. Its an experiment and not a reccomendation!
This article is the first in a series about UDF with Kotlin Flows.
For an interactive tutorial on this topic, check out part 2 of this series:
For a real world app case study with a Spotify clone, check out part 3:
UDF implementations
The goal of an UDF implementation is to represent the effects of a user’s actions with a UI state. The representation of this UI state is open ended, some opt to do this in a single encapsulating data class, others choose to do this with fields on a class body. The latter has some novel implementations, Fabio Collini demonstrated one using Jetpack Compose’s mutableStateOf functions here:
In the case of the former where a single data class is used to encapsulate the entirety of UI state, given that a user may take multiple actions over time, with each action resulting in one or more changes to state, one way of summarizing a UDF implementation is with the following:
val udfImplementation: (Flow<Action>) -> Flow<UiState> = ...
A UDF implementation may be defined as a function that transforms a stream of actions into a stream of state
The above, while concise, is rather dense. It says that a UDF implementation is essentially a function that takes a stream of action and produces a stream of state. What I love about this statement is how applicable it is to existing UDF implementations.
Mobius
public static void main(String[] args) { // Let's make a Mobius Loop MobiusLoop<Integer, CounterEvent, CounterEffect> loop = Mobius .loop(new CounterLogic(), new CounterEffectHandler()) .startFrom(0); // And start using our loop loop.dispatchEvent(CounterEvent.INCREMENT); // Model is now 1 loop.dispatchEvent(CounterEvent.DECREMENT); // Model is now 0 loop.dispatchEvent(CounterEvent.DECREMENT); // Sound effect plays! Model is still 0 }
MVIKotlin
internal class CalculatorStoreFactory(private val storeFactory: StoreFactory) { fun create(): CalculatorStore = object : CalculatorStore, Store<Intent, State, Nothing> by storeFactory.create( name = "CounterStore", initialState = State(), reducer = ReducerImpl ) { } private object ReducerImpl : Reducer<State, Intent> { override fun State.reduce(msg: Intent): State = when (msg) { is Intent.Increment -> copy(value = value + 1L) is Intent.Decrement -> copy(value = value - 1L) } } }
MVI with Arkadii Ivanov’s MVIKotlin
Molecule
@Composable fun SomePresenter(events: Flow<EventType>): ModelType { // ... } val models: StateFlow<ModelType> = scope.launchMolecule { SomePresenter(events) }
The implementations above differ in approach, side effect propagation, available levels of abstraction and so on, but they all effectively serve to reduce actions into state.
Any proponent of React’s Redux should find this familiar, the concepts are the same: action goes in, state comes out. Molecule is particularly interesting for reasons well summarized by the team behind it:
The reason this is exciting, and the reason for the history lesson above, is that Compose does enable a new way of writing our logic. The use of a compiler plugin unlocks the language in a way that otherwise could not be achieved with raw coroutine library APIs.
That set off something for me however, a little code challenge. How succinct / easy would it be to write an implementation of (Flow<Action>) -> Flow<State>
with just simple coroutine library APIs?
Mutator as a UDF implementation
A Mutator is an interface declaration specifying an input and its resulting output.
interface Mutator<Action : Any, State : Any> { val state: State val accept: (Action) -> Unit }
A Mutator is a declaration of an input Action to it’s ensuing State output
However, an interface declaration is only as good as its implementation. Most MVI implementations require the use of a Reducer to process each action into state. With Kotlin’s receivers, we can define a generic reducer as a Mutation
.
typealias Mutation<State> = State.() -> State
A generic reducer is a class describing the mutation of the reduction
In the above, rather than define a specific Reducer
for actions, a data class holding a single lambda defining the reduction works just as well.
Next is the fun part. An MVI implementation of the type above with Kotlin Flows would have the type Mutator<Action, StateFlow<State>>
. The above could be easily achieved if we could split the input Action
stream by its type and specify a Flow
of Mutation<State>
for each one. The resulting Flow<Mutation<State>>
for each Action
can then be merged into a Flow<State>
given an initial State
to reduce into.
For example, consider the Jetpack Compose multiplatform desktop app in the image below:
The state in the app is driven by a Mutator
The screen's UI state is defined as:
data class State( val gridSize: Int = 1, val shouldScrollToTop: Boolean = true, val isInNavRail: Boolean = false, val hasFetchedAuthStatus: Boolean = false, val isSignedIn: Boolean = false, val queryState: QueryState, val lastVisibleKey: Any? = null, val items: List<ArchiveItem> = listOf() )
The user can also take a variety of actions that mutate the state, summarized with a sealed class as:
sealed class Action(val key: String) { sealed class Fetch : Action(key = "Fetch") { abstract val query: ArchiveQuery data class Reset(override val query: ArchiveQuery) : Fetch() data class LoadMore(override val query: ArchiveQuery) : Fetch() } data class FilterChanged(val descriptor: Descriptor) : Action(key = "FilterChanged") data class GridSize(val size: Int) : Action(key = "GridSize") data class ToggleFilter(val isExpanded: Boolean? = null) : Action(key = "ToggleFilter") data class LastVisibleKey(val itemKey: Any) : Action(key = "LastVisibleKey") }
This is standard MVI (Model-View-Intent) fare; each Action
takes in the arguments it needs to perform its function, analogous to a regular method invocation. Each Action
then causes its own Mutation
of state. A StateFlow
based Mutator
for the screen can be defined as:
fun archiveListMutator( scope: CoroutineScope, initialState: State? = null, archiveRepository: ArchiveRepository, authRepository: AuthRepository, … ): ArchiveListMutator = stateFlowMutator( scope = scope, initialState = initialState ?: State(...), started = SharingStarted.WhileSubscribed(2_000L), actionTransform = { actions -> merge( …, authRepository.authMutations(), actions.toMutationStream(keySelector = Action::key) { when (val action = type()) { is Action.Fetch -> action.flow.fetchMutations( scope = scope, repo = archiveRepository ) is Action.FilterChanged -> action.flow.filterChangedMutations() is Action.ToggleFilter -> action.flow.filterToggleMutations() is Action.LastVisibleKey -> action.flow.resetScrollMutations() is Action.GridSize -> action.flow.gridSizeMutations() } } ).monitorWhenActive(lifecycleStateFlow) } )
ArchiveMutator definition. Note how each Action is responsible for its own mutations
In the above, each Action
stream is mapped to its own Mutation
stream using the Flow<Action>.toMutationStream()
extension method. The implementations range from the simple:
private fun Flow<Action.GridSize>.gridSizeMutations(): Flow<Mutation<State>> = distinctUntilChanged() .map { Mutation<State> { copy(gridSize = it.size) } }
A simple Action to Mutation stream: distinctUntilChanged, then mapped
to nuanced:
/** * Every toggle isExpanded == null should be processed, however every specific request to * expand or collapse, should be distinct until changed. */ private fun Flow<Action.ToggleFilter>.filterToggleMutations(): Flow<Mutation<State>> = map { it.isExpanded } .scan(listOf<Boolean?>()) { emissions, isExpanded -> (emissions + isExpanded).takeLast(2) } .transformWhile { emissions -> when { emissions.isEmpty() -> Unit emissions.size == 1 -> emit(emissions.first()) else -> { val (previous, current) = emissions if (current == null || current != previous) emit(current) } } true } .map { isExpanded -> Mutation<State> { copy(queryState = queryState.copy(expanded = isExpanded ?: !queryState.expanded)) } }
A more complex Action to Mutation stream: distinctUntilChanged unless null is emitted
The great thing above is each Action is independent and fully responsible for its resultant Mutation<State>
. Using the appropriate Flow
operators, one can:
Process each Action sequentially
Operate on only the most recently emitted
Or come up with whatever processing pipeline is needed on a per
Action
basis. EachAction
subtype can even be split by its subtype for even more complex pipelines.
The source for the app shown above can be found here:
Fun fact, this article you're reading was written and edited with the app linked above!
Imperative vs Functional Programming
Interestingly, transforming each Action
Flow
into a cold Flow<Mutation<State>>
with an extension function ends up mirroring the more conventional and imperative “invoke method to mutate state” paradigm, but in a more static and functional way. The Flow
extensions have no reference to class variables; instead, they describe what changes to apply to the State
at the time they are processed.
Consider the following imperative snippet where UI state is mutated via method calls on a private backing variable when fetching articles for a category:
fun fetchArticles(category: String) { fetchJob?.cancel() fetchJob = viewModelScope.launch { try { val newsItems = repository.newsItemsForCategory(category) _uiState.update { it.copy(newsItems = newsItems) } } catch (ioe: IOException) { // Handle the error and notify the UI when appropriate. _uiState.update { val messages = getMessagesFromThrowable(ioe) it.copy(userMessages = messages) } } } }
The equivalent functional representation of the above as a Flow<Mutation<State>>
of the SetCategory
Action
is:
fun Flow<Action.SetCategory>.categoryChangeMutations( repository: Repository ): Flow<Mutation<NewsUiState>> = mapLatest { (category) -> try { val newsItems = repository.newsItemsForCategory(category) Mutation<NewsUiState> { copy(newsItems = newsItems) } } catch (ioe: IOException) { // Handle the error and notify the UI when appropriate. val messages = getMessagesFromThrowable(ioe) Mutation<NewsUiState> { copy(userMessages = messages) } } }
Notice the use of maplatest
to match the imperative version where a job reference is kept to prevent having two requests in flight.
I personally prefer the functional version because I get to:
Drop the backing
_uiState
variable that I would need to mutate imperativelyDrop the extra
Job?
class variable that prevented accidental simultaneous fetchesDefine the changes to state caused by the
SetCategory
Action
statically. The function has everything it needs to produce itsMutation
, and does not need the call context of a class body to read class fields.
The disadvantages of the above being:
The classic: "readers and maintainers of the code need to be familiar with
Flows
and their operators".Extra object allocation:
For every use of
Action.SetCategory
that the imperative version avoids by simply being a method call.For every
Mutation
createdFor every UI state created after the
Mutation
is applied
In my personal opinion, the benefits outweigh the caveats, leaving what is essentially the redux pattern without the hassle of Reducers
and side effect handlers. That is, it has all the pros and cons of Redux minus boilerplate.
For what it's worth, I don't think functional programming is always better than imperative programming,
I just prefer it here for working on user events/actions as a stream. Imperative code can be a lot easier to write or think about. A suspending function is a lot more readable to me than Single.just()
or Maybe.just()
or whatever the factory function for creating a stream is in your FRP library of choice.
Testing
No architectural pattern exploration is complete without a section on testing. Being able to represent changes to UI state as a result of a particular Action
with a cold Flow
offers a huge idiomatic advantage for asserting the (Flow<Action>) -> Flow<UiState>
maxim.
For reasons why it is desirable to test cold Flows
instead of hot Flows
, especially StateFlow
, consider reading Testing StateFlow Is Annoying by Bill Phillips.
Above, we outlined the nuanced business logic of "distinctUntilChanged unless null" in filterToggleMutations()
for Action.ToggleFilter
. A test to verify its behavior using Cash app's Turbine is:
@Test fun testFilterToggleMutations() = runTest { val initialQueryState = QueryState( expanded = false, startQuery = ArchiveQuery(kind = ArchiveKind.Articles), currentQuery = ArchiveQuery(kind = ArchiveKind.Articles), ) val initialState = State( queryState = initialQueryState ) listOf( Action.ToggleFilter(isExpanded = null), Action.ToggleFilter(isExpanded = null), Action.ToggleFilter(isExpanded = true), Action.ToggleFilter(isExpanded = true), Action.ToggleFilter(isExpanded = true), Action.ToggleFilter(isExpanded = true), Action.ToggleFilter(isExpanded = false), ) .asFlow() .filterToggleMutations() .reduceInto(initialState) .test { // First item is initial state assertEquals( expected = initialState, actual = awaitItem() ) // After processing null for `isExpanded`, the toggle should have flipped assertEquals( expected = initialState.queryState.copy(expanded = true), actual = awaitItem().queryState ) // Toggle expanded again assertEquals( expected = initialState.queryState.copy(expanded = false), actual = awaitItem().queryState ) // Explicit set expanded to true assertEquals( expected = initialState.queryState.copy(expanded = true), actual = awaitItem().queryState ) // Consecutive emissions of true should have been ignored // Explicit set expanded to false assertEquals( expected = initialState.queryState.copy(expanded = false), actual = awaitItem().queryState ) awaitComplete() } }
The above is idiomatic and terse; for a given set of actions, assert the input yielded the expected output.
For Mutation
Flow
pipelines that take arguments, mocks need to only be provided for the arguments the method requires. In the archiveListMutator()
definition above, you may have noticed other Mutation
contributions outside actions taken by the user. Specifically AuthRepository.authMutations()
which is defined as:
internal fun AuthRepository.authMutations(): Flow<Mutation<State>> = isSignedIn .distinctUntilChanged() .map { Mutation { copy( isSignedIn = it, hasFetchedAuthStatus = true, ) } }
A test for this becomes:
@Test fun testAuthMutations() = runTest { val initialState = State(...) val authRepository = mockk<AuthRepository>() every { authRepository.isSignedIn } returns listOf( false, false, true, ).asFlow() authRepository .authMutations() .reduceInto(initialState) .test { assertEquals( expected = initialState, actual = awaitItem() ) assertEquals( expected = initialState.copy(isSignedIn = false), actual = awaitItem() ) assertEquals( expected = initialState.copy(isSignedIn = true), actual = awaitItem() ) awaitComplete() } }
With a Mutator
, not only is the expression (Flow<Action>) -> Flow<UiState>
explicit, testing it also is.
Wrapping up
There are a lot more things to share about my findings from my experiments with Mutator
, particularly those that produce a StateFlow
. From handling UI events, to representing calls that suspend
and return a value. Again, care should be taken to note, the above is not prescriptive. This is one way of several to represent a pipeline of the production of UI state.
The Mutator definition, stateFlowMutator()
function and Flow<T>.toMutationStream{ }
extension function can be found here:
The above being syntactic sugar around a Flow
pipeline as an implementation of the generic UDF function (Flow<Action>) -> Flow<State>
.
As promised, here’s the implementation for splitting a Flow into its subtypes:
/** * Class holding the context of the [Action] emitted that is being split out into * a [Mutation] [Flow]. * * Use typically involves invoking [type] to identify the [Action] stream being transformed, and * subsequently invoking [flow] to perform a custom transformation on the split out [Flow]. */ data class TransformationContext<Action : Any>( private val type: Action, val backing: Flow<Action> ) { /** * A convenience for the backing [Flow] of the [Action] subtype from the parent [Flow] */ inline val <reified Subtype : Action> Subtype.flow: Flow<Subtype> get() = backing as Flow<Subtype> /** * the first [Action] of the specified type emitted from the parent [Flow] */ fun type() = type } /** * Transforms a [Flow] of [Action] to a [Flow] of [Mutation] of [State], allowing for finer grained * transforms on subtypes of [Action]. This allows for certain actions to be processed differently * than others. For example: a certain action may need to only cause mutations on distinct * emissions, whereas other actions may need to use more complex [Flow] transformations like * [Flow.flatMapMerge] and so on. * * [transform]: a function for mapping independent [Flow]s of [Action] to [Flow]s of [State] * [Mutation]s * @see [splitByType] */ fun <Action : Any, State : Any> Flow<Action>.toMutationStream( // Ergonomic hack to simulate multiple receivers transform: TransformationContext<Action>.() -> Flow<Mutation<State>> ): Flow<Mutation<State>> = splitByType( selector = { it }, transform = transform ) /** * Transforms a [Flow] of [Input] to a [Flow] of [Output] by splitting the original into [Flow]s * of type [Selector]. Each independent [Flow] of the [Selector] type can then be transformed * into a [Flow] of [Output]. * * [selector]: The mapping to the type the [Input] [Flow] should be split into * [transform]: a function for mapping independent [Flow]s of [Selector] to [Flow]s of [Output] */ fun <Input : Any, Selector : Any, Output : Any> Flow<Input>.splitByType( selector: (Input) -> Selector, // Ergonomic hack to simulate multiple receivers transform: TransformationContext<Selector>.() -> Flow<Output> ): Flow<Output> = channelFlow<Flow<Output>> mutationFlow@{ val keysToFlowHolders = mutableMapOf<String, FlowHolder<Selector>>() this@splitByType .collect { item -> val mapped = selector(item) val flowKey = mapped::class.qualifiedName ?: throw IllegalArgumentException("Only well defined classes can be split") when (val existingHolder = keysToFlowHolders[flowKey]) { null -> { val holder = FlowHolder(mapped) keysToFlowHolders[flowKey] = holder val emission = TransformationContext(mapped, holder.exposedFlow) val mutationFlow = transform(emission) channel.send(mutationFlow) } else -> { // Wait for downstream to be connected existingHolder.internalSharedFlow.subscriptionCount.first { it > 0 } existingHolder.internalSharedFlow.emit(mapped) } } } } .flatMapMerge( concurrency = Int.MAX_VALUE, transform = { it } ) fun <State : Any> Flow<Mutation<State>>.reduceInto(initialState: State): Flow<State> = scan(initialState) { state, mutation -> mutation.mutate(state) } /** * Container for representing a [Flow] of a subtype of [Action] that has been split out from * a [Flow] of [Action] */ private data class FlowHolder<Action>( val firstEmission: Action, ) { val internalSharedFlow: MutableSharedFlow<Action> = MutableSharedFlow() val exposedFlow: Flow<Action> = internalSharedFlow.onStart { emit(firstEmission) } }