Kotlin Delegation by Inception
Delegating to delegates with a functional twist
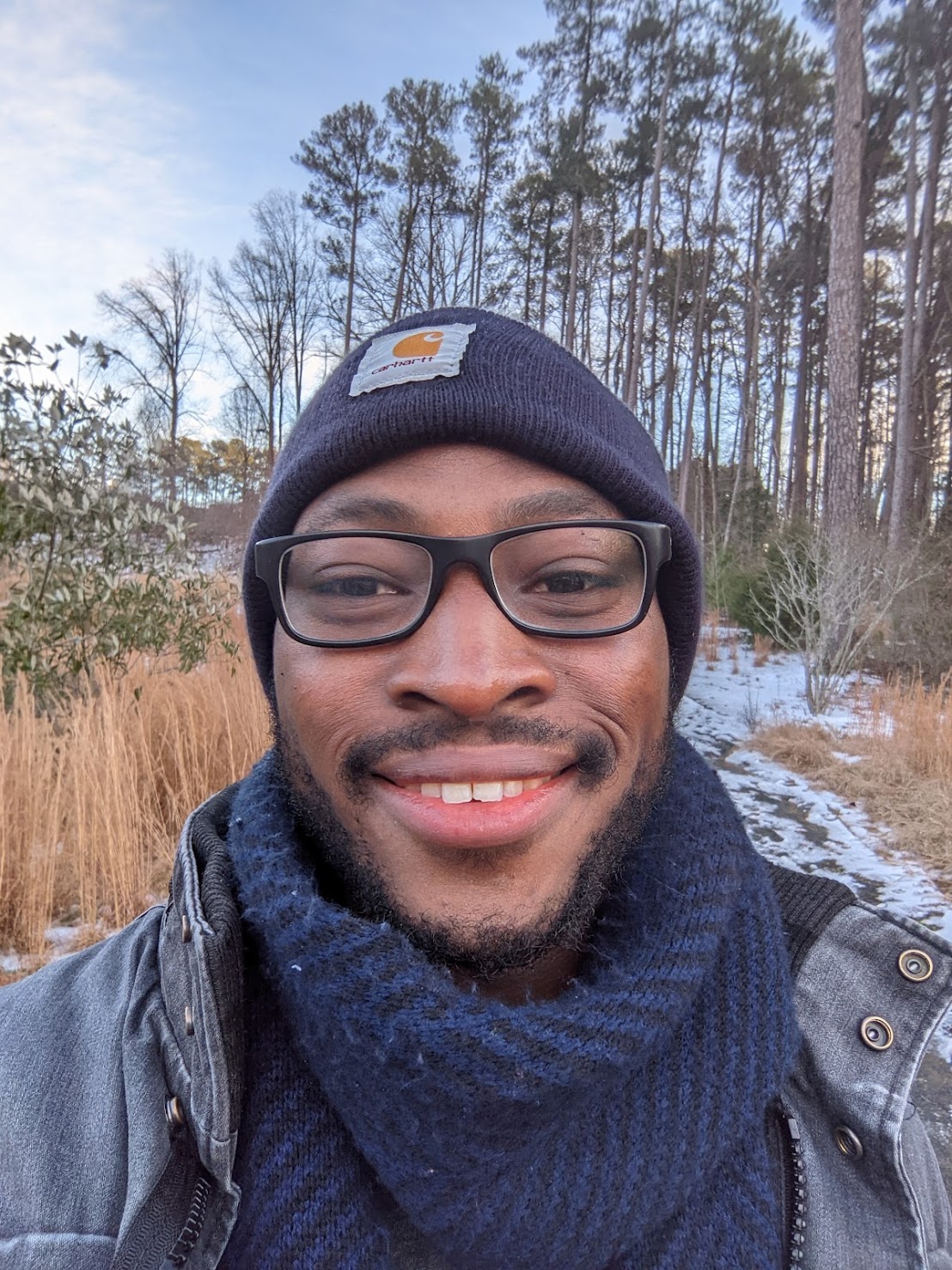
TJ Dahunsi
Dec 12 2020 · 6 min read
I think Kotlin delegates are underused; they are the best implementation of the “favor composition over inheritance” refrain.
1data class ListContainer(val backing: List<Int>): List<Int> by backing
In the above, the ListContainer
is a List
that can be iterated through by delegating to the backing List
within itself. Delegates
are so much more than that however; fundamentally a Delegate
allows for a property to have its read and/or write semantics implemented by an arbitrary bit of code. One of the most underutilized, and therefore the least learned from in my opinion, is the map()
Delegate
.
1class User(val map: MutableMap<String, Any?>) { 2 var name: String by map 3 var age: Int by map 4}
A class whose properties delegate to and therefore mutate the backing map passed to it
In the above, read/writes to the User
instance are delegated to the backing map
; changes in the fields are reflected in the map
immediately. Although a neat example, most Android apps don’t have data blobs marshaled in maps
, they have it in Bundles
. A more useful Delegate
Android wise therefore would be:
1 2private class BundleDelegate<T>( 3 private val default: T? = null 4) : ReadWriteProperty<Bundle, T> { 5 @Suppress("UNCHECKED_CAST") 6 override operator fun getValue( 7 thisRef: Bundle, 8 property: KProperty<*> 9 ): T = when (val value = thisRef.get(property.name)) { 10 null -> default 11 else -> value 12 } as T 13 14 override fun setValue(thisRef: Bundle, property: KProperty<*>, value: T) = 15 thisRef.putAll(bundleOf(property.name to value)) 16}
A Delegate for reading/writing from and to an Android Bundle
This would let you write the following expressions to let you read/write from a Bundle
without having to declare extra string constants all over the place, the key is simply the property name:
1 2var Bundle.booleanProperty by BundleDelegate(true) 3var Bundle.numberProperty by BundleDelegate(1) 4var Bundle.stringProperty by BundleDelegate("Hi") 5var Bundle.parcelableProperty by BundleDelegate<NsdServiceInfo?>()
The above is nice, but not very flexible as it’s an extension on the Bundle
type itself. The more interesting usages of Bundles
in Android tend to be via proxy; Intent
extras, Activity
deep link params and Fragment
arguments all internally delegate to a Bundle
instance. What would be really nice is if we could write a Delegate
that itself delegated to something else that provides a type we already know how to delegate to; or delegation by inception as I like to call it:
1 2private class MappedDelegate<In, Out, T>( 3 private val source: ReadWriteProperty<In, T>, 4 private val postWrite: ((Out, In) -> Unit)? = null, 5 private val mapper: (Out) -> In 6) : ReadWriteProperty<Out, T> { 7 8 override fun getValue(thisRef: Out, property: KProperty<*>): T = 9 source.getValue(mapper(thisRef), property) 10 11 override fun setValue(thisRef: Out, property: KProperty<*>, value: T) { 12 val mapped = mapper(thisRef) 13 source.setValue(mapped, property, value) 14 postWrite?.invoke(thisRef, mapped) 15 } 16} 17 18fun <In, Out, T> ReadWriteProperty<In, T>.map( 19 postWrite: ((Out, In) -> Unit)? = null, 20 mapper: (Out) -> In 21): ReadWriteProperty<Out, T> = 22 MappedDelegate(source = this, postWrite = postWrite, mapper = mapper)
A Delegate whose implementation delegates to another Delegate via a mapper transform
With the above, we can compose Delegates
to arbitrarily read and write to any type, provided the type has a reference to another type that has a ready to use Delegate
. So for Intents
, Activities
and Fragments
we can write:
1 2fun <T> bundleDelegate(default: T? = null): ReadWriteProperty<Bundle, T> = 3 BundleDelegate(default) 4 5fun <T> intentExtras(default: T? = null): ReadWriteProperty<Intent, T> = bundleDelegate(default).map( 6 postWrite = Intent::replaceExtras, 7 mapper = Intent::ensureExtras 8) 9 10fun <T> activityIntent(default: T? = null): ReadWriteProperty<Activity, T> = intentExtras(default).map( 11 postWrite = Activity::setIntent, 12 mapper = Activity::getIntent 13) 14 15fun <T> fragmentArgs(): ReadWriteProperty<Fragment, T> = bundleDelegate<T>().map( 16 mapper = Fragment::ensureArgs 17) 18 19fun <T> Bundle.asDelegate(default: T? = null): ReadWriteProperty<Any?, T> = bundleDelegate(default).map( 20 mapper = { this } 21) 22 23private val Intent.ensureExtras get() = extras ?: putExtras(Bundle()).let { extras!! } 24 25private val Fragment.ensureArgs get() = arguments ?: Bundle().also(::setArguments)
Delegates for various Android types that use Bundles to marshal data
With this Bundles
become so much more convenient to work with:
1class MainActivity : AppCompatActivity() { 2 ... 3 private val deepLinkTab by activityIntent<Int?>(-1) 4} 5 6class DoggoFragment : Fragment(R.layout.fragment_image_detail) { 7 ... 8 private var doggo: Doggo by fragmentArgs() 9} 10 11data class UserBlob(val bundle: Bundle) { 12 val firstName by bundle.asDelegate<String>() 13 val lastName by bundle.asDelegate<String>() 14 val age by bundle.asDelegate<Int>() 15}
Examples of Android Delegates that all rely on a Bundle
With this, a full User edit flow using the new FragmentResult API may look something like this:
1@Parcelize 2class UserBlob constructor( 3 val bundle: Bundle = Bundle(), 4 firstName: String? = null, 5 lastName: String? = null, 6 age: Int? = null 7): Parcelable { 8 9 var firstName by bundle.asDelegate(firstName) 10 private set 11 var lastName by bundle.asDelegate(lastName) 12 private set 13 var age by bundle.asDelegate(age) 14 private set 15 16 init { 17 // Only necessary bc the default value is only used if no existing value is present. 18 firstName?.let(this::firstName::set) 19 lastName?.let(this::lastName::set) 20 age?.let(this::age::set) 21 } 22 23 companion object { 24 val EDITED = "UserEdited" 25 } 26} 27 28class UserViewFragment : Fragment() { 29 override fun onCreate(savedInstanceState: Bundle?) { 30 super.onCreate(savedInstanceState) 31 parentFragmentManager 32 .setFragmentResultListener(UserBlob.EDITED, this) { _, bundle -> 33 viewModel.postUser(UserBlob(bundle)) 34 } 35 } 36} 37 38class UserEditFragment : Fragment() { 39 private var existingUser by fragmentArgs<UserBlob>() 40 41 override fun onResume() { 42 super.onResume() 43 val newUser = UserBlob(existingUser.bundle, firstName = "Blake") 44 parentFragmentManager 45 .setFragmentResult(UserBlob.EDITED, newUser.bundle) 46 } 47 48 companion object { 49 fun newInstance(userBlob: UserBlob) = 50 UserEditFragment().apply { this.existingUser = userBlob } 51 } 52}
In the above, the edited user has their name set to “Blake”, wile keeping the existing user’s last name and age. Also in UserEditFragment
, the user data will survive process death since it is stored in the arguments bundle; all this with no bundle.getParcelable(“propertyKey”)
, or fragment.arguments.getParcelable(“userKey”)
in sight.
We need to go deeper
Why stop there though? I wrote recently on how ViewBinding
makes it really easy to express Views
as a function of their State. In situations like that, it often is very helpful if the View
could remember the last bit of state it was bound to; typically to memoize animations. Now all Android View
instances let you save arbitrary bits of data in them via their setTag
and getTag
methods with unique integer resource ids. If this is making you start thinking of a map
like Delegate
for a View
that took full advantage of this, you’re in luck:
1 2private class ViewDelegate< T>( 3 private val default: T? = null, 4) : ReadWriteProperty<View, T> { 5 @Suppress("UNCHECKED_CAST") 6 override operator fun getValue(thisRef: View, property: KProperty<*>): T { 7 val map = thisRef 8 .getOrPutTag<MutableMap<String, Any?>>(R.id.view_delegate_property_map, ::mutableMapOf) 9 return (map[property.name] ?: default) as T 10 } 11 12 override fun setValue(thisRef: View, property: KProperty<*>, value: T) { 13 val map = thisRef 14 .getOrPutTag<MutableMap<String, Any?>>(R.id.view_delegate_property_map, ::mutableMapOf) 15 map[property.name] = value 16 } 17} 18 19inline fun <reified T> View.getOrPutTag(@IdRes id: Int, initializer: () -> T) = 20 getTag(id) as? T ?: initializer().also { setTag(id, it) }
A delegate for a View to store arbitrary types via its tags
Much like with Bundles
above, if we have any class that has a reference to a View
, we can write delegates for it that internally delegate to it:
1fun <T> viewDelegate(default: T? = null): ReadWriteProperty<View, T> = 2 ViewDelegate(default) 3 4fun <T> viewBindingDelegate(default: T? = null): ReadWriteProperty<ViewBinding, T> = 5 viewDelegate(default).map(mapper = ViewBinding::getRoot) 6 7fun <T> viewHolderDelegate(default: T? = null): ReadWriteProperty<RecyclerView.ViewHolder, T> = 8 viewDelegate(default).map(mapper = RecyclerView.ViewHolder::itemView)
Delegates for a View, ViewBinding and even a RecyclerView ViewHolder
Again, just like before with Intents
, Activities
and Fragments
; any ViewBinding
or RecyclerView.ViewHolder
instance can have any arbitrary property. This is especially useful for:
-
RecyclerView.ViewHolder
instances, because it means you don’t ever need to subclass it again; just addprivate
var
properties that delegate to the type you want. This is the whole foundation for declarativeRecyclerViews
covered here. -
ViewBinding
orView
instances needing a custom animator. In the below, aTextView
has a customObjectAnimator
tightly coupled with it without needing to subclass it.
1private val TextView.textColorAnimator by viewDelegate( 2 ValueAnimator.ofObject(ArgbEvaluator(), Color.RED) 3 .setDuration(400L) 4) 5 6var TextView.animatedTextColor 7 get() = textColorAnimator.animatedValue as? Int ?: currentTextColor 8 set(value) { 9 textColorAnimator.apply { 10 cancel() 11 setIntValues(currentTextColor, value) 12 start() 13 } 14 }
Using a private delegate to strongly couple an ObjectAnimator with a TextView
The possibilities are quite endless. JSON deserialization is one of the most common things an app has to do and libraries often use reflection at runtime to help with it. With the right Delegate
however, you could declare fields in a class and read the value from a common JSON type without the need for reflection.
In summary, Kotlin delegates are one of the best features of the language, and seem to be woefully underused. In your codebase there’s probably some utility function that does a transform that a Delegate
is better suited for. Why not add replacing it with a custom Delegate
to your New Year’s resolutions?
All the aforementioned Delegates
are bundled (pun intended) in the following dependency, and more reading about how Kotlin Delegates
under the hood can be found here.
The delegates described above and more can be found here:
12