Home Automation With Android Things & Kotlin
My Android Things Home Automation Hub, the green dongle is the TI CC2531
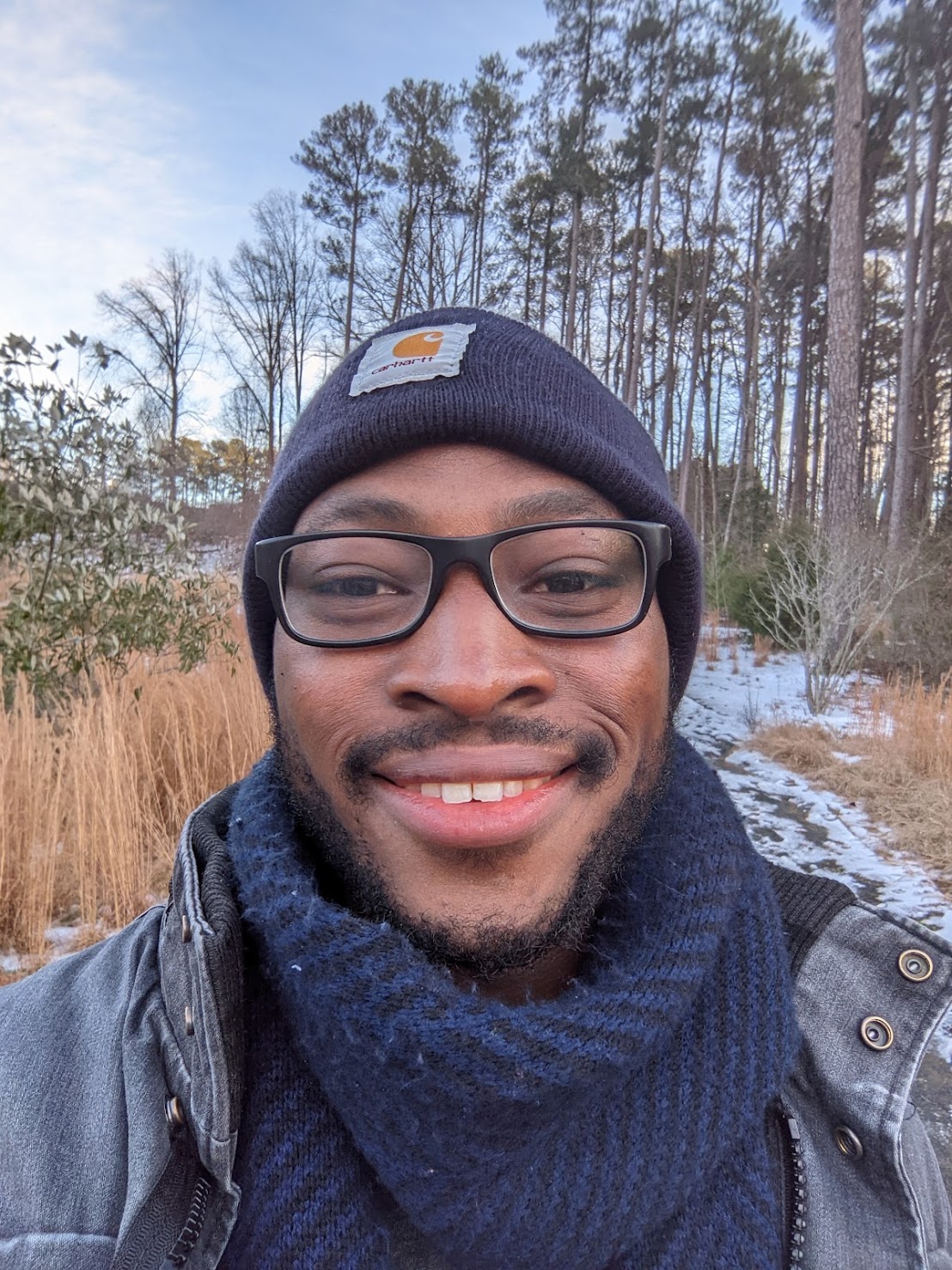
TJ Dahunsi
Jul 08 2019 · 7 mins
We’re spoiled for choice these days when it comes to choosing a Home Automation system. We could go voice controlled with Alexa or Google Assistant, or choose from well established ecosystems like that from Phillip’s Hue. All these systems have one thing in common, proprietary hubs that act as the center of our smart homes. Some more extensible than others, however it’d be nice if we could build our own hubs independent of these proprietary systems and administer them as we wish.
Android is an extremely versatile operating system with APIs for a smattering range of technologies used in IOT from Bluetooth to WiFi, along with USB host mode to interface with other peripherals quite conveniently, making it a great candidate to build a Home Automation hub with. If that weren’t enough, Android Things is even less stringent than Android on phones and tablets as it eschews permission prompts for Bluetooth and USB, implicitly granting them on request on the device it runs on. So, let’s start cooking.
Parts & Supplies
Raspberry Pi 3, please do not get the B+, only the B is supported for Android Things ($35).
SD Card with Android Things image, at least 16 GB ($5)
Rf Transmitter and Receiver for RF modules ($7)
RF wall plugs from Etekcity (3 pack $24)
Texas Instruments CC2531 ZigBee Dongle ($14)
CC Debugger to flash CC2531 ($15) the official one from Texas Instruments costs $50, but you can find generic ones like that linked above for less. If you don’t mind springing more, I’d advise to get the official debugger.
Gledopto multicolor ZigBee Bulb ($25). Hue bulbs should work too, but a Hue bulb is almost twice the price!
Optional:
Server-Client Liaisons
First, there needs to be a way for client devices (Android phones owned by you or your guests) to send instructions to the hub. Since this is a home automation system, let’s keep things local; Android supports Network Service Discovery or NSD, which is a fantastic way for realtime communications with devices on the same local network. It’s based on DNS-SD which is how wireless printing works on a LAN. Essentially each client will open a socket on the hub, and write instructions to it directly, and receive responses on that same socket allowing for duplex communication.
private class ServerThread internal constructor(private val serverSocket: ServerSocket) : Thread(), Closeable { @Volatile internal var isRunning: Boolean = false private val portMap = ConcurrentHashMap<Int, Connection>() private val protocol = ProxyProtocol(ConsoleWriter(this::broadcastToClients)) private val pool = Executors.newFixedThreadPool(5) override fun run() { isRunning = true Log.d(TAG, "ServerSocket Created, awaiting connections.") while (isRunning) { try { Connection( // Create new connection for every new client serverSocket.accept(), // Block this ServerThread till a socket connects this::onClientWrite, this::broadcastToClients, this::onConnectionOpened, this::onConnectionClosed) .apply { pool.submit(this) } } catch (e: Exception) { Log.e(TAG, "Error creating client connection: ", e) } } Log.d(TAG, "ServerSocket Dead.") } private fun onConnectionOpened(port: Int, connection: Connection) { portMap[port] = connection Log.d(TAG, "Client connected. Number of clients: ${portMap.size}") } private fun onConnectionClosed(port: Int) { portMap.remove(port) Log.d(TAG, "Client left. Number of clients: ${portMap.size}") } private fun onClientWrite(input: String?): String { Log.d(TAG, "Read from client stream: $input") return protocol.processInput(input).serialize() } @Synchronized private fun broadcastToClients(output: String) { Log.d(TAG, "Writing to all connections: ${JSONObject(output).toString(4)}") pool.execute { portMap.values.forEach { it.outWriter.println(output) } } } override fun close() { isRunning = false for (key in portMap.keys) catcher(TAG, "Closing server connection with id $key") { portMap[key]?.close() } portMap.clear() catcher(TAG, "Closing server socket.") { serverSocket.close() } catcher(TAG, "Shutting down execution pool.") { pool.shutdown() } } }
ServerThread managing concurrent communication with multiple clients
Note that the SocketThread is blocked waiting for a client to connect. This however doesn’t stop clients from writing back to the server as the other methods are called on their own separate threads.
/** * Connection between [ServerNsdService] and it's clients */ private class Connection internal constructor( private val socket: Socket, private val inputProcessor: (input: String?) -> String, private val outputProcessor: (output: String) -> Unit, private val onOpen: (port: Int, connection: Connection) -> Unit, private val onClose: (port: Int) -> Unit ) : Runnable, Closeable { val port: Int = socket.port lateinit var outWriter: PrintWriter override fun run() { if (!socket.isConnected) return onOpen.invoke(port, this) try { outWriter = createPrintWriter(socket) val reader = createBufferedReader(socket) // Initiate conversation with client outputProcessor.invoke(inputProcessor.invoke(CommsProtocol.PING)) while (true) { val input = reader.readLine() ?: break val output = inputProcessor.invoke(input) outputProcessor.invoke(output) if (output == "Bye.") break } } catch (e: IOException) { e.printStackTrace() } finally { try { close() } catch (e: IOException) { e.printStackTrace() } } } @Throws(IOException::class) override fun close() { onClose.invoke(port) socket.close() } }
Connection class managing IO between the Server and its clients
Client side, the class responsible for managing the socket is the ClientThread
.
private class ClientThread internal constructor( internal var service: NsdServiceInfo, internal var clientNsdService: ClientNsdService) : Thread(), Closeable { var currentSocket: Socket? = null private var out: PrintWriter? = null private val pool = Executors.newSingleThreadExecutor() override fun run() = try { Log.d(TAG, "Initializing client-side socket. Host: " + service.host + ", Port: " + service.port) currentSocket = Socket(service.host, service.port) out = createPrintWriter(currentSocket) val `in` = createBufferedReader(currentSocket) Log.d(TAG, "Connection-side socket initialized.") clientNsdService.connectionState = ACTION_SOCKET_CONNECTED if (!clientNsdService.messageQueue.isEmpty()) { out?.println(clientNsdService.messageQueue.remove()) } while (true) { val fromServer = `in`.readLine() ?: break Log.i(TAG, "Server: $fromServer") val serverResponse = Intent() serverResponse.action = ACTION_SERVER_RESPONSE serverResponse.putExtra(DATA_SERVER_RESPONSE, fromServer) Broadcaster.push(serverResponse) if (fromServer == "Bye.") { clientNsdService.connectionState = ACTION_SOCKET_DISCONNECTED break } } } catch (e: IOException) { e.printStackTrace() clientNsdService.connectionState = ACTION_SOCKET_DISCONNECTED } finally { close() } internal fun send(message: String) { out?.let { if (it.checkError()) close() else pool.submit { it.println(message) } } } override fun close() { App.catcher(TAG, "Exiting message thread") { currentSocket?.close() } clientNsdService.connectionState = ACTION_SOCKET_DISCONNECTED } }
Note again that the client uses a separate ExecutorService to send messages to the server, while the main thread itself manages the socket opened between it and the server and broadcasts any server responses using the Broadcaster
class which is just a wrapper around an Rx PublishProcessor
.
Both the ServerThread
and ClientThread
are hosted in instances of Android Service
classes to keep them running in the background.
Since communication will be over a raw input and output streams, there needs to be a a method of serializing and deserializing each communication Payload
. We can use a simple Kotlin data class of the same name to do this:
data class Payload(val key: String) : Serializable { var data: String? = null var action: String? = null var response: String? = null val commands = LinkedHashSet<String>() fun addCommand(command: String) = commands.add(command) }
Simple Payload class
Next we need an abstract way for our hub to communicate with whatever peripherals it may care about, both synchronously to respond directly to client requests, and asynchronously if a peripheral broadcasts an update. Synchronously, our previous Payload
data class class works, a Payload
comes in as input, is processed, and an output Payload
goes out. For asynchronous commands, each instance of a CommsProtocol
class has a PrintWriter
it can directly write out of. It’s important that whatever is written out is a serialized representation of a Payload
, else the client won’t be able to interpret it.
abstract class CommsProtocol internal constructor(internal val printWriter: PrintWriter) : Closeable { val appContext: Context = App.instance abstract fun processInput(payload: Payload): Payload fun processInput(input: String?): Payload = processInput(when (input) { null, PING -> Payload(CommsProtocol::class.java.name).apply { action = PING } RESET -> Payload(CommsProtocol::class.java.name).apply { action = RESET } else -> input.deserialize(Payload::class) }) protected fun getString(@StringRes id: Int): String = appContext.getString(id) protected fun getString(@StringRes id: Int, vararg args: Any): String = appContext.getString(id, *args) companion object { const val PING = "Ping" internal const val RESET = "Reset" val sharedPool: ExecutorService = Executors.newFixedThreadPool(5) } }
The Base class for responding to client request server side
Server-Peripheral Liaisons
Now that data can be sent to and received from the client, implementations of the CommsProtocol can be written. Simple RF wall sockets and ZigBee bulbs cover my basic home automation needs for now, and so that’s all I have implemented, however, the system is designed to be extensible to support whatever peripheral.
SerialRFPRotocol
Android Things unfortunately, is quite slow, making it impossible to interface directly with RF transceivers and essentially duplicate the packets sent out by cheap wireless switches. An Arduino however, is perfect for this, and it’s as easy as adding a USB serial dependency to use serial over USB to communicate with an Arduino; and that’s exactly what this protocol does. It sends commands to the Arduino to sniff switches, and when the packet sniffed, it replicates it letting you use your phone as a universal remote. The video below is the original implementation that uses BLE instead of a wired serial connection, which still works but is a bit over engineered and contrived. I’ve since moved to a direct wired connection.
ZigBeeProtocol
This protocol creates a ZigBee network that ZigBee peripherals can join. It again uses Serial over USB to communicate with Texas Instruments’ CC2531 dongle running their Z-STACK ZigBee 3.0 solution. All of the heavy lifting is done by ZSmartSystems’ ZigBee Cluster library. It essentially ports their CLI application to an Android GUI along with conveniences for switching light bulbs on and off. The real fun part was writing an implementations of their ZigBeePort
for Android. Again it uses the excellent USB Serial for Android library for managing serial communications. A video of its operation is shown below.
So, that’s one way to build a home automation hub for a little under $100. Full source follows: