Declarative lists on Android With RecyclerView + ViewBinding
One liner xml to stateful RecyclerView.ViewHolder instance
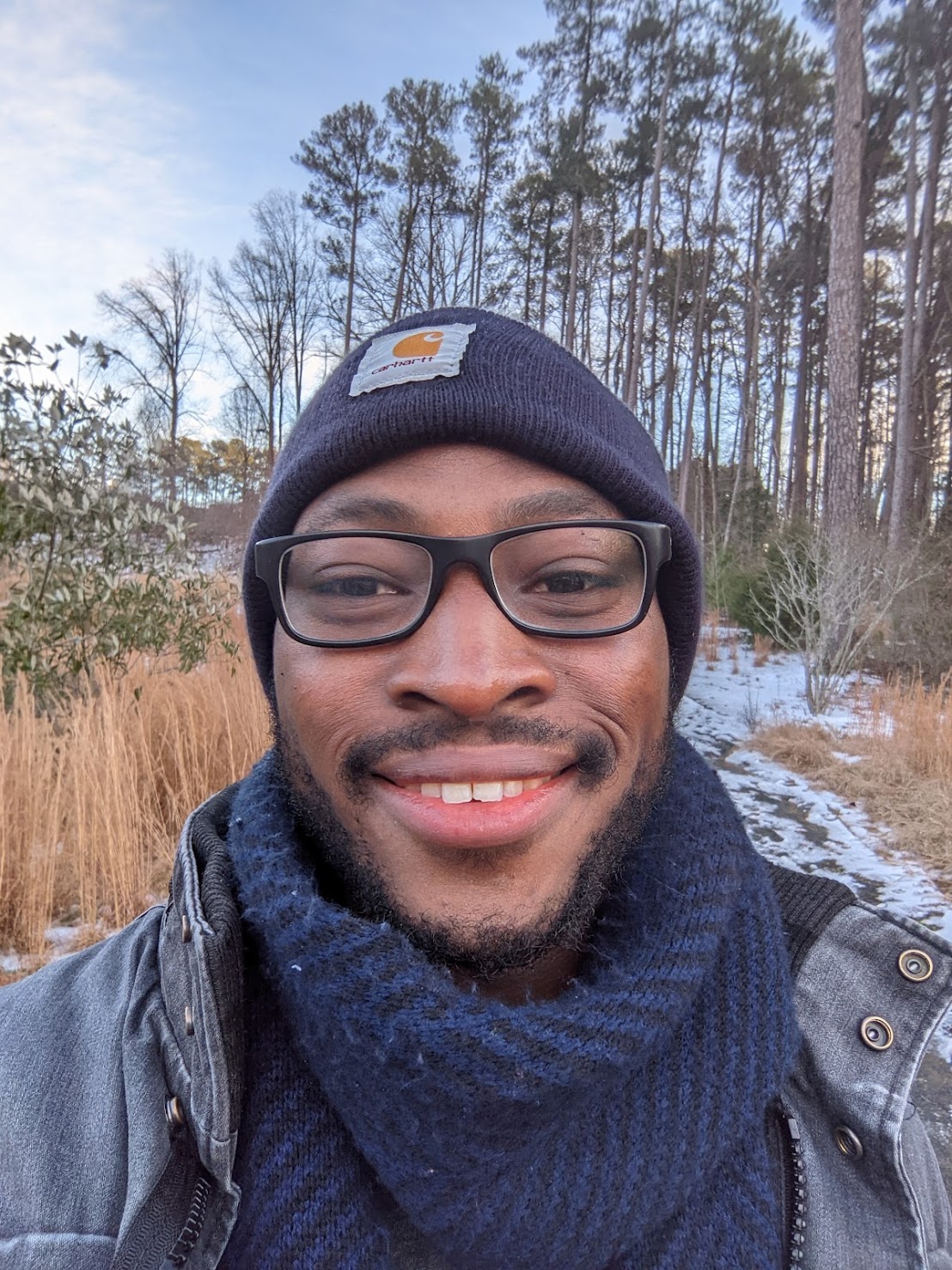
TJ Dahunsi
Mar 01 2020 · 7 mins
The RecyclerView
is an extremely flexible and indispensable Android ViewGroup
. It unfortunately requires quite a bit of boilerplate to set up; a fully functional RecyclerView
asks that you:
Set a
LayoutManager
Create an
Adapter
to return the different kinds of view types you may haveSpecify
ViewHolder
classes for each of the different view typesImplement binding for each of them
That’s at least two classes that need to subclassed for every list shown and gets tedious very, very quickly.
Write once, use everywhere
In the case of the Adapter
, one remedy is to create a method that takes individual arguments that maps to each public RecyclerView.Adapter
method, where the only arguments required are the necessities like those for creating and binding ViewHolders
. This allows for composing an Adapter
so it becomes completely unnecessary to extend the base RecyclerView.Adapter class anymore.
private class ComposedAdapter<ItemT : Any?, VH : RecyclerView.ViewHolder>( private val itemsSource: () -> List<ItemT>, private val viewHolderCreator: (ViewGroup, Int) -> VH, private val viewHolderBinder: (holder: VH, item: ItemT, position: Int) -> Unit, private val viewTypeFunction: ((ItemT) -> Int)? = null, private val itemIdFunction: ((ItemT) -> Long)? = null, ... /* other callbacks follow */ ) : RecyclerView.Adapter<VH>() { init { setHasStableIds(itemIdFunction != null) } override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): VH = viewHolderCreator(parent, viewType) override fun onBindViewHolder(holder: VH, position: Int) = viewHolderBinder(holder, itemsSource()[position], position) override fun getItemCount(): Int = itemsSource().size override fun getItemViewType(position: Int): Int = viewTypeFunction?.invoke(itemsSource()[position]) ?: super.getItemViewType(position) override fun getItemId(position: Int): Long = itemIdFunction?.invoke(itemsSource()[position]) ?: super.getItemId(position) override fun onBindViewHolder(holder: VH, position: Int, payloads: MutableList<Any>) = viewHolderPartialBinder?.invoke(holder, itemsSource()[position], position, payloads) ?: super.onBindViewHolder(holder, position, payloads) ... /* ... and so on */ }
Breaking out all the callbacks for a RecyclerView Adapter out to allow for easy composition
A version for composing a ListAdapter also exists here: tunjid/Android-Extensions An Android library with modules to quickly bootstrap an Android application. - tunjid/Android-Extensionsgithub.com
Enter ViewBinding
With Android Studio 3.6’s stable release, we can use ViewBinding
to further cut down on boilerplate with respect to ViewHolder
creation as well, as fundamentally there is little difference between the intent behind the view holder pattern, and a view binding, i.e they are both wrapper classes around one or more View
instances.
First, taking a look at the APIs, A RecyclerView.ViewHolder
has a reference to its root itemView
. Similarly, all generated ViewBinding
classes inherit from the ViewBinding
interface with the following signature:
/** A type which binds the views in a layout XML to fields. */ public interface ViewBinding { /** * Returns the outermost {@link View} in the associated layout file. If this binding is for a * {@code <merge>} layout, this will return the first view inside of the merge tag. */ @NonNull View getRoot(); }
From a view property perspective, the APIs are identical. Peeking further into generated ViewBinding
classes like outlined in Mark Allison’s Styling Android post, the following generated static methods can be seen to exist on every ViewBinding
instance:
public final class MyViewHolderBinding implements ViewBinding { @NonNull private final ConstraintLayout rootView; @NonNull public final TextView text1; private MyViewHolderBinding(@NonNull ConstraintLayout rootView, @NonNull TextView text1) { this.rootView = rootView; this.text1 = text1; } @Override @NonNull public ConstraintLayout getRoot() { return rootView; } @NonNull public static MyViewHolderBinding inflate(@NonNull LayoutInflater inflater) { ... } @NonNull public static MyViewHolderBinding inflate(@NonNull LayoutInflater inflater, @Nullable ViewGroup parent, boolean attachToParent) { ... } @NonNull public static MyViewHolderBinding bind(@NonNull View rootView) { ... }
The great thing here is the static inflate(@NonNull LayoutInflater inflater, @Nullable ViewGroup parent, boolean attachToParent)
method. Its signature is nearly identical to that used in the onCreateViewHolder
for a RecyclerView.Adapter
: it takes a parent ViewGroup
and inflates a View
using the LayoutParams of the parent, and crucially doesn’t attach it to the parent ViewGroup
. Attachment is left to the RecyclerView
to do when it’s ready to add the ViewHolder
to the list, and when it wants to detach it for recycling.
Taking advantage of this, We can create a BindingViewHolder
class generic on ViewBinding
type, that holds a reference to the ViewBinding
, not just the itemView
.
open class BindingViewHolder<T : ViewBinding> private constructor( val binding: T ) : RecyclerView.ViewHolder(binding.root) { constructor( parent: ViewGroup, creator: (inflater: LayoutInflater, root: ViewGroup, attachToRoot: Boolean) -> T ) : this(creator( LayoutInflater.from(parent.context), parent, false )) } fun <T : ViewBinding> ViewGroup.viewHolderFrom( creator: (inflater: LayoutInflater, root: ViewGroup, attachToRoot: Boolean) -> T ): BindingViewHolder<T> = BindingViewHolder(this, creator)
So if you have an existing XML file that you intend to use in a ViewHolder, creating a ViewHolder instance is as simple simple as passing the method references of the static inflate
method and calling:
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): BindingViewHolder<MyViewHolderBinding> = parent.viewHolderFrom(MyViewHolderBinding::inflate)
XML to ViewHolder in a clean 1 liner using a method reference to the static inflate method
Going further with dynamic property binding
Despite this, creating a ViewHolder
is only part of the way to a fully working RecyclerView
implementation. ViewHolders
are often bound to model objects so that when a child View
is interacted with, clicked for example, a callback like a View.OnClickedListener
is invoked and some action performed. A ViewHolder
wrapper around a ViewBinding
can only take us so far, a BindingViewHolder
should also allow the seamless binding of generic properties without having to subclass it again, otherwise, we could just directly subclass RecyclerView.ViewHolder
, and pass it the ViewBinding
reference directly.
This yet again is another example where Kotlin shines as a language because of how malleable it is to a developer’s intent: the general idea is to create a delegate on a BindingViewHolder
that can statically store and retrieve any property of any type on the ViewHolder
while not holding a strong reference to it that can cause a memory leak. In other words a generic function <T>(BindingViewHolder<VB>) -> T
that can be used as a delegate.
The Android View
class via its getTag(@resourceId id: Int)
and setTag(@resourceId id: Int, item: Any?)
API is pretty much equivalent to a Map<Int, Any>
interface. The only caveat here is that the key must be an Android resource Id to reduce the likelihood of hash collisions internally, and if the resource is private, make it almost impossible to leak it outside the module the id is created and used in. We can (ab?)use this however in the following fashion:
open class BindingViewHolder<T : ViewBinding> private constructor( val binding: T ) : RecyclerView.ViewHolder(binding.root) { ... class Prop<T> : ReadWriteProperty<BindingViewHolder<*>, T> { override fun getValue(thisRef: BindingViewHolder<*>, property: KProperty<*>): T { @Suppress("UNCHECKED_CAST") return thisRef.propertyMap[property.name] as T } override fun setValue(thisRef: BindingViewHolder<*>, property: KProperty<*>, value: T) { thisRef.propertyMap[property.name] = value } } } @Suppress("UNCHECKED_CAST") private inline val BindingViewHolder<*>.propertyMap get() { val existing = itemView.getTag(R.id.recyclerview_view_binding_map) as? MutableMap<String, Any?> val delegateMap = existing ?: mutableMapOf<String, Any?>() .also { itemView.setTag(R.id.recyclerview_view_binding_map, it) } return delegateMap }
With the power of Kotlin delegate, we delegate to the root View
of the ViewBinding
instance in the BindingViewHolder
(which is the same reference of the itemView
of the same) to store a reference to a MutableMap<String, Any?>
that is lazily initialized. When attempting to read a property from the BindingViewHolder
, this Map
is read from, and likewise when written to. This makes it possible to declare properties on any BindingViewHolder<T>
of any ViewBinding
that can be written to in onBindViewHolder
of the Adapter
, and read from when an View.OnClickListener
is invoked.
You also needn’t worry if this is inefficient; looking up a View’s
tag is implemented internally with a SparseArray
which uses binary search for lookups, the MutableMap
is constant time, and the delegate implementation is identical to the by Map
delegate in the Kotlin Standard Library. If the extension method that uses the Prop
delegate is defined in a static
context, an extra benefit of having only one Prop
delegate instance being created for the app, inlining the code for the lookup.
How significant is all this? The following is the full implementation of a RecyclerView
that shows the result of a Bluetooth Low Energy scan in a Fragment
.
class BleScanFragment : AppBaseFragment(R.layout.fragment_ble_scan) { private val viewModel by viewModels<BleViewModel>() override fun onViewCreated(view: View, savedInstanceState: Bundle?) { super.onViewCreated(view, savedInstanceState) val recyclerView = FragmentBleScanBinding.bind(view).list.apply { layoutManager = verticalLayoutManager() adapter = adapterOf( itemsSource = viewModel::scanResults, viewHolderCreator = { parent, _ -> parent.viewHolderFrom(ViewholderScanBinding::inflate).apply { itemView.setOnClickListener { onBluetoothDeviceClicked(result.device) } } }, viewHolderBinder = { viewHolder, scanResult, _ -> viewHolder.bind(scanResult) } ) viewModel.devices.observe(viewLifecycleOwner) { diffResult -> recyclerView.adapter?.let { diffResult.dispatchUpdatesTo(it) } } } } } private fun onBluetoothDeviceClicked(bluetoothDevice: BluetoothDevice) { uiState = uiState.copy(snackbarText = bluetoothDevice.address) } } private var BindingViewHolder<ViewholderScanBinding>.result by BindingViewHolder.Prop<ScanResultCompat>() fun BindingViewHolder<ViewholderScanBinding>.bind(result: ScanResultCompat) { this.result = result if (result.scanRecord != null) binding.apply { deviceName.text = result.scanRecord!!.deviceName deviceAddress.text = result.device.address } }
Easy, simple, clean, and extremely convenient. No subclasses of RecyclerView.Adapter
or RecyclerView.ViewHolder
are necessary, all with a declarative API.
Even more stark is the reduction in the number of lines of code for the implementation of the RecyclerView
, I’ll let the following git diff illustrate this:
Added and changed code for BindingViewHolder and static property extension
Deleted code for previous standalone ViewHolder class
This motif is used throughout the Android-Extensions project linked below if you want more examples that include shared element transitions, or animations: