Tiler
A kotlin multiplatform, reactive map-like abstract data type for fetching keyed data
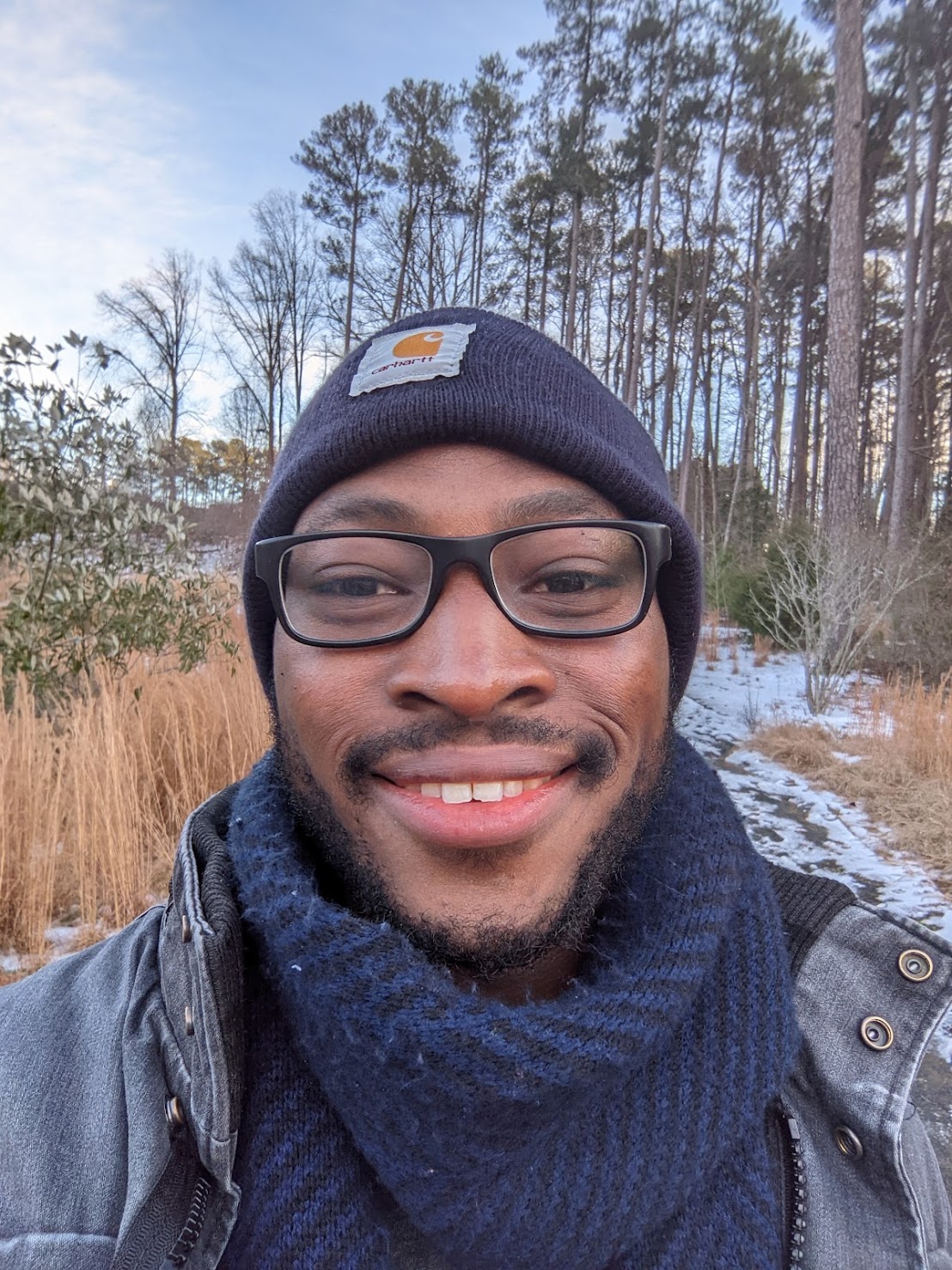
TJ Dahunsi
Feb 27 2022 ยท 2 min read
Tiling is a kotlin multiplatform experiment for loading chunks of structured data from reactive sources.
Tiling is achieved with a Tiler; a pure function with an API most similar to a reactive Map
where:
- The keys are queries for data
- The values are dynamic sets of data returned over time as the result of the query key.
The output of a Tiler is either a snapshot of the Map<Key, Value>
or a flattened List<Value>
making a Tiler
either a:
(Flow<Tile.Input.List<Query, Item>>) -> Flow<List<Item>>
or
(Flow<Tile.Input.Map<Query, Item>>) -> Flow<Map<Query, Item>>
Getting your data
Tilers are implemented as plain functions. Given a Flow
of Input
, you can either choose to get your data as:
- A
Flow<List<Item>>
withtiledList
- A
Flow<Map<Query, Item>>
withtiledMap
In the simplest case given a MutableStateFlow<Tile.Request<Int, List<Int>>
one can write:
1class NumberFetcher { 2 private val requests = 3 MutableStateFlow<Tile.Input.List<Int, List<Int>>>(Tile.Request.On(query = 0)) 4 5 private val tiledList: (Flow<Tile.Input<Int, List<Int>>>) -> Flow<List<List<Int>>> = tiledList( 6 order = Tile.Order.Sorted(comparator = Int::compareTo), 7 fetcher = { page -> 8 val start = page * 50 9 val numbers = start.until(start + 50) 10 flowOf(numbers.toList()) 11 } 12 ) 13 14 val listItems: Flow<List<Int>> = tiledList.invoke(requests).map { it.flatten() } 15 16 fun fetchPage(page: Int) { 17 requests.value = Tile.Request.On(page) 18 } 19}
The above will return a full list of every item requested sorted in ascending order of the pages.
Note that in the above, the list will grow indefinitely as more tiles are requested unless queries are evicted.
This may be fine for small lists, but as the list size grows, some items may need to be evicted and
only a small subset of items need to be presented to the UI. This sort of behavior can be achieved using
the Evict
Request
, and the PivotSorted
Order
covered on the project github page:
0