Animating ContentScale during image shared element transitions
A Jetpack Compose twist on an old favorite
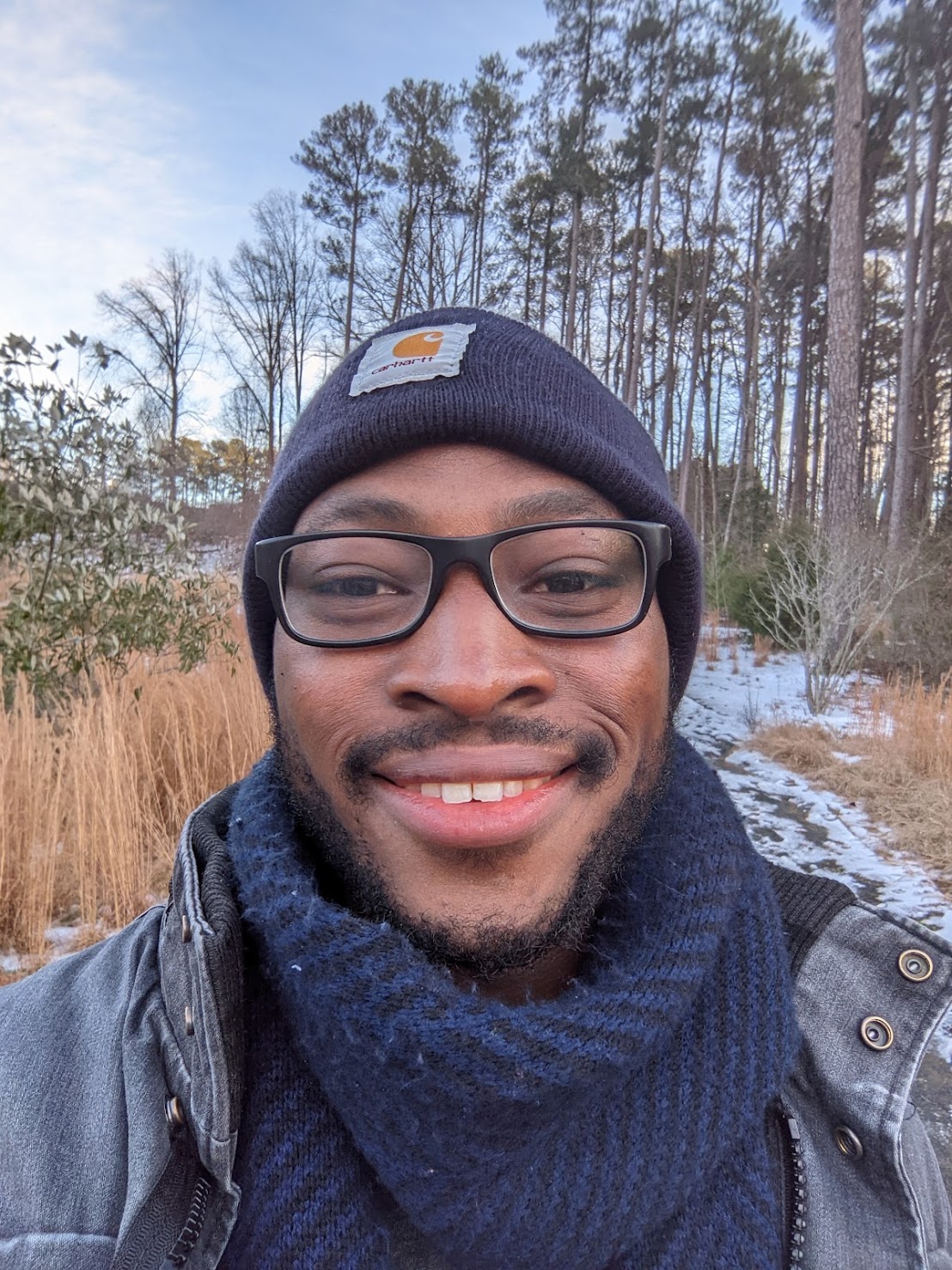
TJ Dahunsi
Mar 21 2024 · 12 mins
I'm obsessed with animations in apps. They provide a sense of place, grant visual continuity and greatly improve the user experience. For details as to why, read this evergreen blog post about building smarter animations with motional intelligence.
Shared element transitions today
This sense of place and continuity is brought to the fore for navigation animations in apps with shared elements, especially with media. To simplify things, this blog will restrict media to just images. Shared elements in the Android View system rely on a smoke and mirrors effect to create the illusion that an element is moving from one destination to the next. It goes something like this:
Screen A passes the size and position of the shared element to screen B.
Screen B reviews the information passed to it and matches its own views to the shared elements.
Screen B then places the matching views where they used to be on Screen A.
Screen B finally animates them to their final position.
This generally works fine, however there are few things that bother me about this:
There are 2 views created that render the same information with minor differences, if any at all.
If the image fetched is asynchronous, and the image has had a transformation applied (different aspect ratio for example); you may have to use
Fragment.postponeEnterTransition()
to postpone the transition and wait till the image on screen B is loaded. After loading is complete,Fragment.startPostponedEnterTransition()
may be used to resume the transition. This interruption can cause jank.If the image is being loaded in a different aspect ratio (
ContentScale
), there's still a mild visual discontinuity as the size and position of the shared element is animated, but theContentScale
is not.
Can Jetpack Compose improve on this?
Thinking differently with Jetpack Compose
I LOVE Jetpack Compose. It requires a new mental model, but with it comes new, and often better ways to approach and solve existing problems. Specifically, with the problem defined above, there are a few neat APIs we can take advantage of to have the closest literal implementation of the concept of a shared element transition on any platform.
To do this, we'll go a little deeper and build upon the foundational APIs for shared elements in Compose that power shared elements. The official guide linked above offers high level APIs for shared elements:
SharedTransitionLayout
: The outermost layout required to implement shared element transitions. It provides a SharedTransitionScope. Composables need to be in a SharedTransitionScope to use the shared element modifiers.Modifier.sharedElement
: The modifier that flags to theSharedTransitionScope
the composable that should be matched with another composable.Modifier.sharedBounds
: The modifier that flags to theSharedTransitionScope
that this composable's bounds should be used as the container bounds for where the transition should take place. In contrast to sharedElement(), sharedBounds() is designed for visually different content.
These work well for 90% of use cases, but it has the some limitations. This post is focused on ContentScale
for images not being animated by default; it snaps to the set end ContentScale
of the image and this can break immersion.
To implement this, we'll need to look at the lower level APIs powering the shared element transitions to create a custom Modifier that maintains immersion and preserved continuity for images even when the ContentScale
changes. The end result is the following:
API overview
The general Compose APIs used in the gif above are:
TargetBasedAnimation
via the animate method.Side effect APIs like
LaunchedEffect
andproduceState
to precisely time when animations should be run.moveableContentOf
: To move UI in composition from one place to another.
The lower level shared element APIs are:
LookaheadScope
: To perform lookahead measurements to guide moving elements to their destination. Notably,SharedTransitionScope
extendsLookaheadScope
.ApproachLayoutModifierNode
: Implementation Modifier.Node used to guide elements that have moved to their destination after lookahead.GraphicsLayer.record
: Used to record drawing commands into aGraphicsLayer
and render them elsewhere.
When assembled, the step by step breakdown is:
A single shared
LookaheadScope
at the root of the navigation hierarchy implemented with aSharedTransitionLayout
.Screen A to use a
moveableContentOf
Composable with anApproachLayoutModifierNode
attached to render its shared element.Screen A to signal navigation to Screen B.
The navigation state to be read on both screens.
Screen A to immediately stop composing the moveable shared element.
Screen B to immediately start composing the shared element.
The animation APIs to gradually animate the size, position AND the
ContentScale
of the shared element to the destination.
Interpolating ContentScale
Interpolating ContentScale
in Compose is as simple as using the linear interpolation lerp
function. To do so, a Composable can be written that remembers and updates the current ContentScale
, and updates the previous ContentScale
whenever the current one changes.
When a change occurs, an animation is launched to drive the interpolation fraction from 0f
to 1f
. Then an anonymous ContentScale
that reads this fraction is finally returned to act as the real ContentScale
in the composition.
@Composable private fun ContentScale.interpolate(): ContentScale { var interpolation by remember { mutableFloatStateOf(1f) } var previousScale by remember { mutableStateOf(this) } val currentScale by remember { mutableStateOf(this) }.apply { if (value != this@interpolate) previousScale = when { interpolation == 1f -> value else -> CapturedContentScale( capturedInterpolation = interpolation, previousScale = previousScale, currentScale = value ) }.also { interpolation = 0f } value = this@interpolate } LaunchedEffect(currentScale) { animate( initialValue = 0f, targetValue = 1f, animationSpec = spring( stiffness = Spring.StiffnessMedium ), block = { progress, _ -> interpolation = progress }, ) } return remember { object : ContentScale { override fun computeScaleFactor( srcSize: Size, dstSize: Size ): ScaleFactor { val start = previousScale.computeScaleFactor( srcSize = srcSize, dstSize = dstSize ) val stop = currentScale.computeScaleFactor( srcSize = srcSize, dstSize = dstSize ) return if (start == stop) stop else lerp( start = start, stop = stop, fraction = interpolation ) } } } }
If for any reason the interpolation animation is interrupted before it completes, an intermediate ContentScale
that captures the interpolation progress is created and set as the current ContentScale and the process continues as normal:
private class CapturedContentScale( private val capturedInterpolation: Float, private val previousScale: ContentScale, private val currentScale: ContentScale, ) : ContentScale { override fun computeScaleFactor( srcSize: Size, dstSize: Size ): ScaleFactor = lerp( start = previousScale.computeScaleFactor( srcSize = srcSize, dstSize = dstSize ), stop = currentScale.computeScaleFactor( srcSize = srcSize, dstSize = dstSize ), fraction = capturedInterpolation ) }
This can be put together for the demo screen below:
Moving shared elements between navigation destinations
Now that ContentScale
can be interpolated, the next step is actually sharing a Composable across navigation destinations while preserving its existing state. More succintly, I'd like to perform a Composable transplant.
The definition that follows is the Composable I want to move:
data class ImageArgs( val url: String?, val description: String? = null, val contentScale: ContentScale ) @Composable fun Image( ImageArgs: ImageArgs, modifier: Modifier ) { Box(modifier) { AsyncImage( modifier = modifier, model = ImageArgs.url, contentDescription = null, contentScale = ImageArgs.contentScale.interpolate() ) } }
Next I create a custom scope at the root of my navigation hierarchy to store my shared elements:
@Stable interface AdaptiveContentState { val navigationState: Adaptive.NavigationState val overlays: Collection<SharedElementOverlay> }
Where a SharedElementOverlay
is the shared element itself:
interface SharedElementOverlay { fun ContentDrawScope.drawInOverlay() }
The actual implementation of AdaptiveContentState
treats navigation as state, and stores the shared elements in a MutableStateMap
.
@Stable class SavedStateAdaptiveContentState @AssistedInject constructor( ... @Assisted saveableStateHolder: SaveableStateHolder, ) : AdaptiveContentState, SaveableStateHolder by saveableStateHolder { override val overlays: Collection<SharedElementOverlay> get() = keysToSharedElements.values private val keysToSharedElements = mutableStateMapOf<Any, SharedElementData<*>>() fun <T> createOrUpdateSharedElement( key: Any, sharedElement: @Composable (T, Modifier) -> Unit, ): @Composable (T, Modifier) -> Unit { val sharedElementData = keysToSharedElements.getOrPut(key) { SharedElementData( sharedElement = sharedElement, onRemoved = { keysToSharedElements.remove(key) } ) } // Can't really guarantee that the caller will use the same key for the right type return sharedElementData.moveableSharedElement } }
The SavedStateAdaptiveContentState
has a method for creating or updating shared elements that may be moved in between Composables by retaining the Composable in a SharedElementData
class. This class is what hosts the moveableContentOf
and keeps track of it's state:
internal class SharedElementData<T>( sharedElement: @Composable (T, Modifier) -> Unit, onRemoved: () -> Unit ) : SharedElementOverlay { val moveableSharedElement: @Composable (Any?, Modifier) -> Unit = movableContentOf { state, modifier -> @Suppress("UNCHECKED_CAST") sharedElement( // The shared element composable will be created by the first screen and reused by // subsequent screens. This updates the state from other screens so changes are seen. state as T, Modifier .movableSharedElement( sharedElementData = this, ) then modifier, ) ... } }
Creating a custom shared element modifier
This is the fun part. So far, the system desgin allows for moving a Composable between screen level Composables, but not for animating it. To animate it, the approachLayout
Modifier is used. Inside the SharedElementData
state are DeferredTargetAnimation
instances for animating the size ind position of the Composable that are self managed. When these animations are running, the Composable can be said to be performing a shared element transition. However this is not the only condition to be met, otherwise the Composables will animate when scrolled and not track with the rest of the UI. To solve this, the Composable watches the navigation state, and allows for animating each time the navigation state changes up until the first time the animations complete:
private fun SharedElementData<*>.isInProgress( animationMapper: (SharedElementData<*>) -> DeferredTargetAnimation<*, *> ): Boolean { val animation = remember { animationMapper(this) } val containerState = LocalAdaptiveContentScope.current ?.containerState ?.also(::updateContainerStateSeen) val (laggingScopeKey, animationInProgressTillFirstIdle) = produceState( initialValue = Pair( containerState?.key, containerState.canAnimateOnStartingFrames() ), key1 = containerState ) { value = Pair( containerState?.key, containerState.canAnimateOnStartingFrames() ) value = snapshotFlow { animation.isIdle } .filter(true::equals) .first() .let { value.first to false } }.value return when(laggingScopeKey == containerState?.key) { true -> animationInProgressTillFirstIdle && hasBeenShared() false -> containerState.canAnimateOnStartingFrames() } }
With this, the custom shared element modifier for moveable shared elements can be defined:
internal fun Modifier.movableSharedElement( sharedElementData: SharedElementData<*>, ): Modifier = composed { val sharedTransitionScope = LocalSharedTransitionScope.current val coroutineScope = rememberCoroutineScope() val sizeAnimInProgress = sharedElementData.isInProgress( SharedElementData<*>::sizeAnimation ) .also { sharedElementData.sizeAnimInProgress = it } val offsetAnimInProgress = sharedElementData.isInProgress( SharedElementData<*>::offsetAnimation ) .also { sharedElementData.offsetAnimInProgress = it } val layer = rememberGraphicsLayer().also { sharedElementData.layer = it } approachLayout( isMeasurementApproachInProgress = { sharedElementData.sizeAnimation.updateTarget( target = it, coroutineScope = coroutineScope, animationSpec = sizeSpec ) sizeAnimInProgress }, isPlacementApproachInProgress = { val target = with(sharedTransitionScope) { lookaheadScopeCoordinates.localLookaheadPositionOf(it) } sharedElementData.offsetAnimation.updateTarget( target = target.round(), coroutineScope = coroutineScope, animationSpec = offsetSpec, ) offsetAnimInProgress }, approachMeasure = { measurable, _ -> val (width, height) = sharedElementData.sizeAnimation.updateTarget( target = lookaheadSize, coroutineScope = coroutineScope, animationSpec = sizeSpec ) val animatedConstraints = Constraints.fixed(width, height) val placeable = measurable.measure(animatedConstraints) layout(placeable.width, placeable.height) layout@{ val currentCoordinates = coordinates ?: return@layout placeable.place( x = 0, y = 0 ) val targetOffset = with(sharedTransitionScope) { lookaheadScopeCoordinates.localLookaheadPositionOf( currentCoordinates ) } sharedElementData.targetOffset = sharedElementData.offsetAnimation.updateTarget( target = targetOffset.round(), coroutineScope = coroutineScope, animationSpec = offsetSpec ) val currentOffset = with(sharedTransitionScope) { lookaheadScopeCoordinates.localPositionOf( sourceCoordinates = currentCoordinates, relativeToSource = Offset.Zero ).round() } val (x, y) = sharedElementData.targetOffset - currentOffset placeable.place( x = x, y = y ) } } ) .drawWithContent { layer.record { this@drawWithContent.drawContent() } if (!sharedElementData.canDrawInOverlay) { drawLayer(layer) } } }
Rendering in overlays
When the shared element transition is in progress, the shared content has to be rendered above other content so they're not occuluded. To do this, Modifier.drawWithContent
is used along with GraphicsLayer.record()
to preserve drawing instructions into the GraphicsLayer
. If the shared element is not transitioning, its simply rendered in place:
drawWithContent { layer.record { this@drawWithContent.drawContent() } if (!sharedElementData.canDrawInOverlay) { drawLayer(layer) } }
If it is transitioning however, it should be drawn at the topmost level in the hierarchy, that is where the SharedTransitionLayout
is defined:
@Composable fun AdaptiveContentRoot( adaptiveContentState: AdaptiveContentState, content: @Composable () -> Unit ) { SharedTransitionLayout( modifier = Modifier.drawWithContent { drawContent() adaptiveContentState.overlays.forEach { overlay -> with(overlay) { drawInOverlay() } } } ) { CompositionLocalProvider(LocalSharedTransitionScope provides this) { content() } } }
As previously mentioned, these overlays are the SharedElementData
from before:
@Stable internal class SharedElementData<T>( sharedElement: @Composable (T, Modifier) -> Unit, onRemoved: () -> Unit ) : SharedElementOverlay { private var layer: GraphicsLayer? = null private var sizeAnimInProgress by mutableStateOf(false) private var offsetAnimInProgress by mutableStateOf(false) private val canDrawInOverlay get() = sizeAnimInProgress || offsetAnimInProgress val offsetAnimation = DeferredTargetAnimation( vectorConverter = IntOffset.VectorConverter, ) val sizeAnimation = DeferredTargetAnimation( vectorConverter = IntSize.VectorConverter, ) val moveableSharedElement: @Composable (Any?, Modifier) -> Unit = ... override fun ContentDrawScope.drawInOverlay() { if (!canDrawInOverlay) return val overlayLayer = layer ?: return val (x, y) = targetOffset.toOffset() translate(x, y) { drawLayer(overlayLayer) } } }
Wrapping up
In the above, the shared element state is preserved across both screens. i.e; it is a literal shared element. No dual views, no smoke and mirrors, no postponing and starting transitions. Just well designed and aptly named Jetpack Compose APIs doing exactly what they say on the tin. I still find it mind blowing that this is even possible. This is one of those things that I'm not even sure where I'd start to do this with Views.
Thinking outside the Box() {}
So that was cool. Let's push on a bit.
Predictive Back
Predictive back in Android is a cool feature that lets users see where they're going when they perform the back gesture without committing to it. Since we're pushing boundaries, let's assume that apps can have 1 - N navigation destinations active at any one time.
Using the setup described above, a skeuomorphic predictive back navigation transition of quite literally popping a navigation destination off the top of a stack can be created, while still having the shared element fall to the lower destination that will end up at the top.
Large screens
You're not sated? Perhaps you're even unimpressed. Maybe 2 simultaneous navigation destinations isn't impressive. Fair shout. May I interest you in 3? With the shared element transition still working?
Declarative APIs for Declarative UIs
The above is part of a series of experiments I have under the working title "Declarative APIs for Declarative UIs".
The basic argument is, Jetpack Compose is amazing and powerful, but it is a significant paradigm shift. Should we also start to rethink the APIs that assemble the state for Compose in the UI layer to get the best from it?
I think the answer is yes, and phrased even stronger: Jetpack Compose requires first frame ready developer readable and writable state production all across the UI layer to truly shine as the next generation UI toolkit that it is.
In the examples above, I needed the following APIs to be first frame ready in the UI layer:
Pagination as state: Paginated data is an immutable
List
subclass that can be written to and read from at any time. For shared elements with navigation, the pagination pipeline can be seeded from navigation arguments and available instantaneously at the point the shared element transition starts. My experiment for this is Tiler.Navigation as state: Navigation data is immutable data hoisted outside the composition and transparently readable and writable anywhere in the application. Changes to navigation are read downstream, and combined with other UI signals like display size, can be dynamically adapted to the visible UI. My experiment for this is treeNav.
A robust UDF state management solution: I personally prefer MVI, and my experiment for this is Mutator.
Each of these will have dedicated blog posts deconstructing how they contributed to the examples shown above, so stay tuned. Also, if you're still on the fence on Compose, you really ought to come on down. It's quite nice here.
The app hosting all these experiments can be found below.